Expand description
Implementation of the ChaCha family of stream ciphers.
Cipher functionality is accessed using traits from re-exported [cipher
] crate.
ChaCha stream ciphers are lightweight and amenable to fast, constant-time implementations in software. It improves upon the previous Salsa design, providing increased per-round diffusion with no cost to performance.
This crate contains the following variants of the ChaCha20 core algorithm:
ChaCha20
: standard IETF variant with 96-bit nonceChaCha8
/ChaCha12
: reduced round variants of ChaCha20XChaCha20
: 192-bit extended nonce variantXChaCha8
/XChaCha12
: reduced round variants of XChaCha20ChaCha20Legacy
: “djb” variant with 64-bit nonce. WARNING: This implementation internally uses 32-bit counter, while the original implementation uses 64-bit counter. In other words, it does not allow encryption of more than 256 GiB of data.
§⚠️ Security Warning: Hazmat!
This crate does not ensure ciphertexts are authentic, which can lead to serious vulnerabilities if used incorrectly!
If in doubt, use the chacha20poly1305
crate instead, which provides
an authenticated mode on top of ChaCha20.
USE AT YOUR OWN RISK!
§Diagram
This diagram illustrates the ChaCha quarter round function. Each round consists of four quarter-rounds:
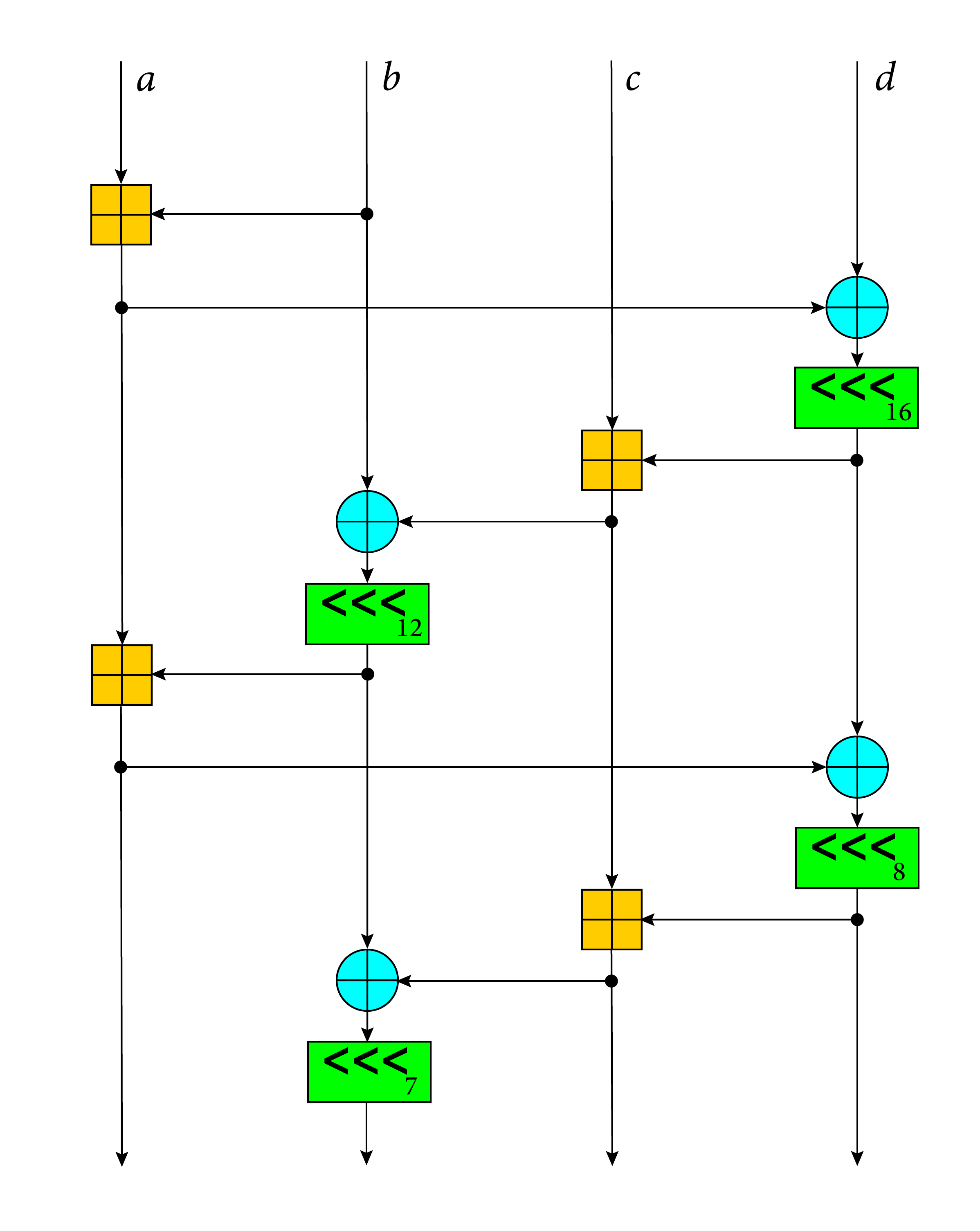
Legend:
- ⊞ add
- ‹‹‹ rotate
- ⊕ xor
§Example
use chacha20::ChaCha20;
// Import relevant traits
use chacha20::cipher::{KeyIvInit, StreamCipher, StreamCipherSeek};
use hex_literal::hex;
let key = [0x42; 32];
let nonce = [0x24; 12];
let plaintext = hex!("00010203 04050607 08090A0B 0C0D0E0F");
let ciphertext = hex!("e405626e 4f1236b3 670ee428 332ea20e");
// Key and IV must be references to the `GenericArray` type.
// Here we use the `Into` trait to convert arrays into it.
let mut cipher = ChaCha20::new(&key.into(), &nonce.into());
let mut buffer = plaintext.clone();
// apply keystream (encrypt)
cipher.apply_keystream(&mut buffer);
assert_eq!(buffer, ciphertext);
let ciphertext = buffer.clone();
// ChaCha ciphers support seeking
cipher.seek(0u32);
// decrypt ciphertext by applying keystream again
cipher.apply_keystream(&mut buffer);
assert_eq!(buffer, plaintext);
// stream ciphers can be used with streaming messages
cipher.seek(0u32);
for chunk in buffer.chunks_mut(3) {
cipher.apply_keystream(chunk);
}
assert_eq!(buffer, ciphertext);
§Configuration Flags
You can modify crate using the following configuration flags:
chacha20_force_avx2
: force AVX2 backend on x86/x86_64 targets. Requires enabled AVX2 target feature. Ignored on non-x86(-64) targets.chacha20_force_neon
: force NEON backend on ARM targets. Requires enabled NEON target feature. Ignored on non-ARM targets. Nightly-only.chacha20_force_soft
: force software backend.chacha20_force_sse2
: force SSE2 backend on x86/x86_64 targets. Requires enabled SSE2 target feature. Ignored on non-x86(-64) targets.
The flags can be enabled using RUSTFLAGS
environmental variable
(e.g. RUSTFLAGS="--cfg chacha20_force_avx2"
) or by modifying .cargo/config
.
You SHOULD NOT enable several force
flags simultaneously.
Re-exports§
pub use cipher;
Structs§
- The ChaCha20 stream cipher (legacy “djb” construction with 64-bit nonce).
- The ChaCha core function.
- The XChaCha core function.
Functions§
- The HChaCha function: adapts the ChaCha core function in the same manner that HSalsa adapts the Salsa function.
Type Aliases§
- ChaCha8 stream cipher (reduced-round variant of
ChaCha20
with 8 rounds) - ChaCha12 stream cipher (reduced-round variant of
ChaCha20
with 12 rounds) - ChaCha20 stream cipher (RFC 8439 version with 96-bit nonce)
- The ChaCha20 stream cipher (legacy “djb” construction with 64-bit nonce).
- Key type used by all ChaCha variants.
- Nonce type used by
ChaCha20Legacy
. - Nonce type used by ChaCha variants.
- XChaCha8 stream cipher (reduced-round variant of
XChaCha20
with 8 rounds) - XChaCha12 stream cipher (reduced-round variant of
XChaCha20
with 12 rounds) - XChaCha is a ChaCha20 variant with an extended 192-bit (24-byte) nonce.
- Nonce type used by XChaCha variants.